Hi @scharifa.bornschier,
I’m sorry for the time it took to come up with an answer.
Here’s a modification of the code you’ve provided (which funny enough, I think I wrote the first one).
from grovepi import *
from grove_rgb_lcd import *
from time import sleep
from time import time
from math import isnan
dht_sensor_port = 7 # connect the DHt sensor to port 7
dht_sensor_type = 1 # change this depending on your sensor type - see header comment
soil_moisture_sensor = 0 # connected to analog port 0 - aka A0
duty_cycle = 0.2 # the percentage of time when the dht is one (0-1 -> 0-100%)
time_window = 4 # the frame of time when both the dht and the moisture sensor are displayed
dht_on = True
moisture_on = False
hourglass_dht = duty_cycle * time_window # amount of time the dht is displayed
hourglass_moisture = (1 - duty_cycle) * time_window # amount of time the moisture sensor is displayed
start_time = time()
setRGB(0,255,255)
def display_dht():
[ temp,hum ] = dht(dht_sensor_port,dht_sensor_type) # get the temperature and Humidity from the DHT sensor
# check if we have nans
# if so, then raise a type error exception
if isnan(temp) is True or isnan(hum) is True:
raise TypeError('nan error')
t = str(temp)
h = str(hum)
# display the dht stats
setText_norefresh("Temp:" + t + "C\n" + "Humidity:" + h + "%")
print("temp = " + str(temp) + "C\thumidity = " + str(hum) + "%")
def display_moisture():
soil_moisture = analogRead(soil_moisture_sensor) # get stats on the soil's moisture
# display the moisture stats
# also add some space after we print the data, so we can clean any unwanted characters
setText_norefresh("Soil\nMoisture: " + str(soil_moisture) + " " * 3)
print("soil moisture = " + str(soil_moisture))
while True:
try:
elapsed_time = time() - start_time
# if we are set to displaying the dht stats then
if dht_on is True:
# check if the hourglass has burned out
if hourglass_dht > elapsed_time:
display_dht()
# but if the hourglass has burned out, then switch over to displaying the moisture stats
else:
# clean the LCD
setText("")
# select the moisture sensor
dht_on = False
moisture_on = True
# reset the hourglass time
start_time = time()
# if otherwise we're set to the moisture sensor
elif moisture_on is True:
# check if the hourglass has burned out
if hourglass_moisture > elapsed_time:
display_moisture()
# but if the hourglass has burned out, then switch over to displaying the dht stats
else:
# clean the LCD
setText("")
# select the dht sensor
dht_on = True
moisture_on = False
# reset the hourglass time
start_time = time()
except (IOError, TypeError) as e:
print(str(e))
# and since we got a type error
# then reset the LCD's text
setText("")
except KeyboardInterrupt as e:
print(str(e))
# since we're exiting the program
# it's better to leave the LCD with a blank text
setText("")
break
I have been testing it and it works really nice.
Here’s a GIF
of the this example program running.
The GIF
appears darker, because the LCD
is really bright, so I had to lower down the brightness.
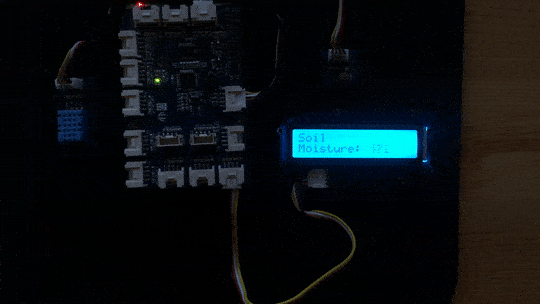
As you can see, the stats for the DHT
sensor are displayed for a lot less time than the moisture sensor.
That’s because there a few lines of code that allows you to determine how much screen time each sensor has.
The variables which are responsible for this are:
-
duty_cycle
- type float
It’s a user set variable which takes values between 0
and 1
and it represents the percentage of time
the DHT
sensor has on the LCD
screen.
The rest of the time is given to the other sensor, which in our case is the moisture
sensor.
This range of 0->1
can be mapped to 0-100%
.
-
time_window
- type float
, measured in seconds
It’s a user set variable which takes any value that’s bigger than 0
and it represents the time in seconds
the DHT
sensor is displayed + the time in seconds
the moisture
sensor is displayed.
Basically, this variable specifies how much time it takes to display both sensors - in a way, it’s kind of refresh rate.
Depending on the application, the duty_cycle
and time_window
variables should be modified.
For instance, in the attached GIF
, the DHT
sensor is displayed for 20%
of the time, whilst the moisture
sensor is displayed for 80%
of the time.
The time it takes to go through all these 2 sensors is specified by the time_window
variable, which in our case is 4
seconds.
This means, that the DHT
sensor is displayed for 20% * 4 seconds = 0.8 seconds
and the moisture
sensor is displayed for 80% * 4 seconds = 3.2 seconds
.
Please, go through the source code and try to understand it - it’s going to help you a lot.
If there’re any other issues or misunderstandings, please let us know.
Thank you!