Hello,
we are using the temperature and humidity Sensor DHT22 - white one, aka DHT Pro or AM2302 and the Grove-LCD RGB Blacklight Display and followed the instruction of the tutorial on Dexter. We also adapted the script for our sensor but the contrast of the text of the display is too low and barely readable, because the backlight is too high and seems to flash all the time.
We used this script:
from grovepi import *
from grove_rgb_lcd import *
dht_sensor_port = 7 # Connect the DHt sensor to port 7
dht_sensor_type = 1 # change this depending on your sensor type - see header comment
while True:
try:
[ temp,hum ] = dht(dht_sensor_port,dht_sensor_type) #Get the temperature and Humidity from the DHT sensor
print("temp =", temp, "C\thumidity =", hum,"%")
t = str(temp)
h = str(hum)
setRGB(0,128,64)
setRGB(0,255,0)
setText("Temp:" + t + "C " + "Humidity :" + h + "%")
except (IOError,TypeError) as e:
print("Error")
The examples of the scripts in the following folder GrovePi/Software/Python/grove_rgb_lcd worked well, so the problem might be with the script.
We don’t know how to continue so please help us!
Hi @scharifa.bornschier,
The continuous flashing is caused by the fact that you’re resetting the LCD
all the time.
A better function for updating the LCD
without having it flash all the time is setText_norefresh(string_to_display)
.
You’re saying the text is barely readable. I think there 2 things that cause this:
-
Because the LCD
is reset in every iteration of the loop - and hence we get that flashing.
-
Because the LCD
is not given some off-time. We should wait some time before re-updating the LCD
screen.
Here’s a code I’ve written for you that works really well.
from grovepi import *
from grove_rgb_lcd import *
from time import sleep
from math import isnan
dht_sensor_port = 7 # connect the DHt sensor to port 7
dht_sensor_type = 1 # use 0 for the blue-colored sensor and 1 for the white-colored sensor
# set green as backlight color
# we need to do it just once
# setting the backlight color once reduces the amount of data transfer over the I2C line
setRGB(0,255,0)
while True:
try:
# get the temperature and Humidity from the DHT sensor
[ temp,hum ] = dht(dht_sensor_port,dht_sensor_type)
print("temp =", temp, "C\thumidity =", hum,"%")
# check if we have nans
# if so, then raise a type error exception
if isnan(temp) is True or isnan(hum) is True:
raise TypeError('nan error')
t = str(temp)
h = str(hum)
# instead of inserting a bunch of whitespace, we can just insert a \n
# we're ensuring that if we get some strange strings on one line, the 2nd one won't be affected
setText_norefresh("Temp:" + t + "C\n" + "Humidity :" + h + "%")
except (IOError, TypeError) as e:
print(str(e))
# and since we got a type error
# then reset the LCD's text
setText("")
except KeyboardInterrupt as e:
print(str(e))
# since we're exiting the program
# it's better to leave the LCD with a blank text
setText("")
break
# wait some time before re-updating the LCD
sleep(0.05)
Please review the code and read the comments I’ve left behind.
Also, here’s a GIF
of my GrovePi
, Grove DHT sensor
and Grove LCD RGB
while using the code I’ve pasted you now.
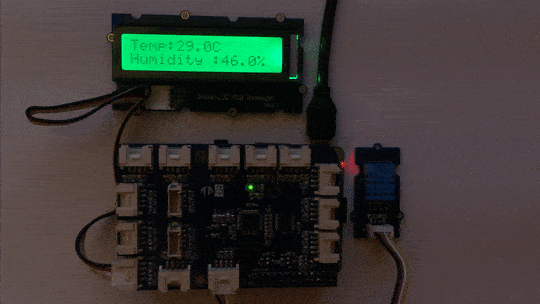
Hope this solves your issue. Let me know if there are more problems with it.
Thank you!
Hi @RobertLucian
Thank you very much for your fast response!
I already adopted your code but there is still a problem with the syntax. In the following there is a picture of the terminal: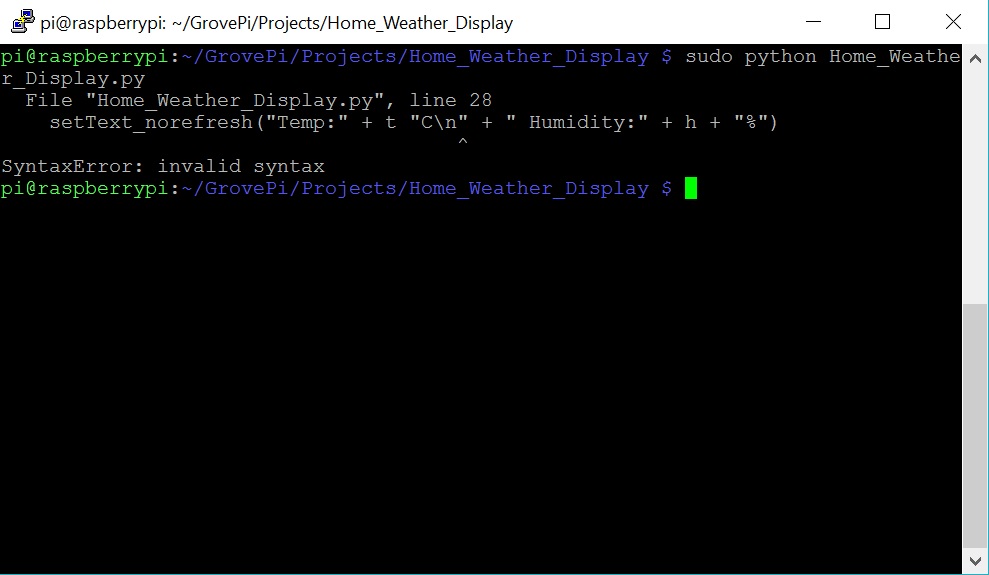
Hope you can also solve this problem.
Thanks a lot!
Hi @scharifa.bornschier,
There’s a plus sign missing.
This is how it should look: "Temp: " + t + "C\n" + "Humidity: " + h +"%"
.
I think you should start with the code from my last post since that one works and then move and build on top of that.
You’ll only need to change the type of sensor (blue
or white
).
How does this sound to you?
I’m looking forward to see if it works.
Thank you!
Hi @RobertLucian
Oh okay i think i was too tired last evening 
I added the plus sign and it’s working!
Thank you so much!
Hi @scharifa.bornschier,
I’m really glad to hear it worked out for you.
I’ve also had moments like this, when I was tired and I didn’t see obvious things. It gets funny when you think about them afterwards.
Can we close the thread? Is there anything else to add?
Thank you!
Hi @RobertLucian
there is nothing to add, so we can close the thread!